qtablewidget pandas dataframe
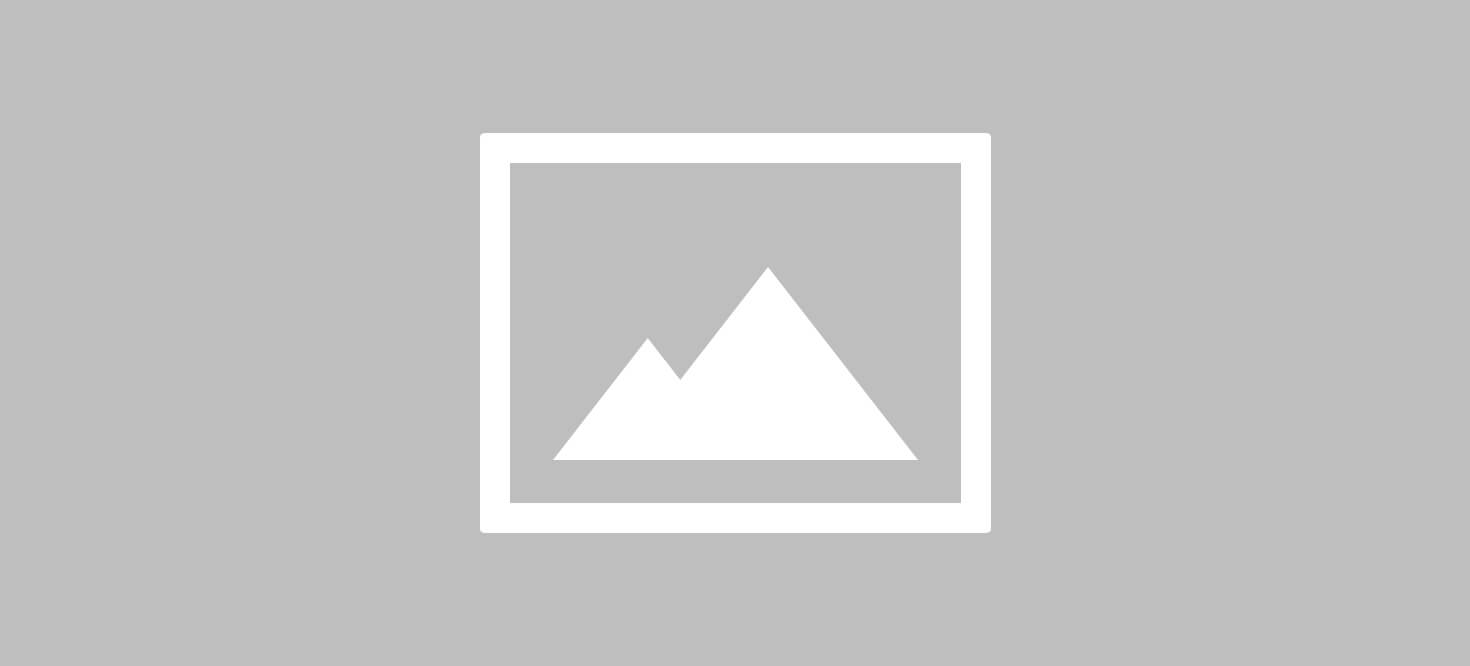
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE At the time of writing this answer, the latest pandas version is 0.18.1 and you could do: That code seems to be coupled to PySide, however it should be relatively trivial to make it work with PyQt. ), setting style properties like text alignment, and even setting color properties for the cell or its content. Q&A: How can I enable editing on a QTableView? Name of this algorithm, and is there a numpy/scipy implementation of it? For more information, please see our Enjoyed this? its official documentation. Here, we use the Pandas str find method to create something like a filter-only column. . The index parameter gives the location in the table for which information is currently being requested, and has two methods .row() and .column() which give the row and column number in the view respectively. m_table) return; const int currentRow = m_table - >rowCount (); m_table - >setRowCount ( currentRow + 1); This takes a data source, for example a list of list objects, a numpy array or a Pandas DataTable and displays it in a Qt table view. If you want to display data arranged in a table, use a QTableWidget to do described below: the reason of using + 1 is to include a new column where pyside """ if role == Qt. With this arrangement when you index, you index first by row, then by column making our example table a 3 row, 5 column table. QtCore.QAbstractTableModel is an abstract base class meaning it does not have implementations for the methods. python pandas matplotlib Here is fully working copy-paste example for PyQT5 # -*- coding: utf-8 -*- # Form implementation generated from reading ui . the simplest way. Iterate the data structure, create the QTableWidgetItems instances, and PythonpandasDataFramepythonpandas.DataFramepandas.DataFrame Excelisin() . Find centralized, trusted content and collaborate around the technologies you use most. We check for role == Qt.TextAlignmentRole and look up the value by index as before, then determine if the value is numeric. Then, you can create an instance of the Table class from the pandastable library and set its model to a TableModel instance created from your Pandas data frame. PyQt5 - Updating DataFrame behind QTableWidget, Efficiently updating NaN's in a pandas dataframe from a prior row & specific columns value, Updating a pandas DataFrame row with a dictionary, Updating dataframe by row but not updating, Pandas DataFrame updating Column values with other DataFrame, Updating a pandas dataframe with a new dataframe, Updating a column in a Pandas DataFrame based on a condition of another column, Select rows from a DataFrame based on a values in another dataframe and updating one of the column with values according to the second DataFrame. Martin Fitzpatrick has been developing Python/Qt apps for 8 years. In Python, Pandas is the. Documentation contributions included herein are the copyrights of their respective owners. This is a simple example of how to display a Pandas data frame with PyQt5/PySide2 using the pandastable library. . Here is the solution to display a Pandas data frame with PyQt5/PySide2 using a QTableWidget: In this code, we first create a Pandas dataframe with some data. QTableWidget () . to use, copy, modify, merge, publish, distribute, sublicense, and/or sell Let's see different methods of formatting integer column of Dataframe in Pandas. The only difference is how we index into the data object. How to iterate over files in directory using Python with example code, How to download Youtube MP3 audio only with Python, How do I check if a list is empty in Python, How to process Excel files in Python with openpyxl. Interested in contributing to the site? In the case of a nested list of list in the arrangement we're using here, the number of rows is simply the number of elements in the outer list, and the number of columns is the number of elements in one of the inner lists assuming they are all equal. For this we just need to go over our data and include the data on a QLineSeries. low high) with a gradient of blue to red. However, you can adapt this as needed, as long as the end result of your handler is to return a QColor or QBrush, QTableView with number-range color gradients. Updating pandas dataframe between processes, Updating element of dataframe while referencing column name and row number, HDFStore updating stored HDF5 python pandas dataframe. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 (https://www.gnu.org/licenses/fdl.html) as published by the Free Software Foundation. In our previous formatting examples we had used text formatting to display float down to 2 decimal places. Implementing the model for our QTableView will allow us to set the headers, manipulate the formats of the cell values (remember we have UTC time and float numbers! You need to handle that yourself! In the mean time you can use, Fastest way to populate QTableView from Pandas data frame, doc.qt.io/qtforpython/examples/example_external__pandas.html, How a top-ranked engineering school reimagined CS curriculum (Ep. Working with tabular data in Python opens up a number of possibilities for how we load and work with that data. The project uses Python port of HierarchicalHeaderView. Note: You can still achieve a similar structure using other Qt elements like QMenuBar, QWidget and QStatusBar, but you will need to take care of the MainWindow layout and design, while QMainWindow already has a layout structure (Right diagram). Matplotlib: setting the figsize very big cause the xtick and yticks hidden. Cookie Notice Here is the final version of our code, remember to download any data and try it out! Following is the snippet of code that reads a CSV file ,create a DataFrame, then display in a GUI: Use Git or checkout with SVN using the web URL. An interesting extension here is to use the table header of the QTableView to display row and pandas column header values, which can be taken from DataFrame.index and DataFrame.columns respectively. QTableWidget . Inside the widget, we create a Table widget from the pandastable library and set its model to a TableModel instance created from the Pandas data frame. Let's look what happens when we execute this code: Note: The output will differ depending on how wide is your screen and which all_hour.csv file you got. What positional accuracy (ie, arc seconds) is necessary to view Saturn, Uranus, beyond? Notice that using a QTableWidget is not the only path to display copies or substantial portions of the Software. This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. Has the DataFrame object from pandas superceded the other alternatives for heterogeneous data types? Code #1 : Round off the column values to two decimal places. How to convert a hex decimal column in scala to int, Join a long data frame to a tidy data frame, Sorting a d.frame by a column of another d.frame R, Is there a way to store pandas dataframe to a Teradata table, Efficient way to build complex dataframe row by row in R, Pass a function with any case class return type as parameter, Iterating each row of Data Frame using pySpark, Pyspark parallelized loop of dataframe column. How To Debug a C++ Extension of a PySide6 Application? Q&A: How can I enable editing on a QTableView? Reducing Django Memory Usage. Enjoyed this? For a better understanding of the concept, we will take an example where we want to display the name and the city of different people in a table in our application. Notice that we first need to check the role is Qt.EditRole to determine if an edit is currently being made. Interested in contributing to the site? If it is, then the handler returns the text (foreground) color red. Your preferences . As in our earlier model view examples, we create the QTableView widget, then create an instance of our custom model (which we've written to accept the data source as a parameter) and then we set the model on the view. In this article, we'll explain how to create Pandas data structure DataFrame Dictionaries . You signed in with another tab or window. Martin Fitzpatrick has been developing Python/Qt apps for 8 years. How to create new dictionaries for a specific key values? to display our data inside. .. versionchanged:: 3.4.0. The first one we will look at it Qgrid from Quantopian. . As you noticed, in the previous step the data was still on a raw state, so now the idea is to select which columns do we need and how to properly handle it. (Item) QTableWidgetItem . In this program I would like to update the QTableview, by setting up a worker thread to process the calculation of new dataframe. Finally, you can add the table widget to the layout and show the main window. We then show the table using table.show() and start the event loop with app.exec_(). Using argparse allow us to have a simple interaction command line interface for our project, so let's take a look how a first attempt You can combine them together by adding them together e.g. excel We can add one or more tables in our PyQt application using QTableWidget. with the class as is I get: for new pandas version you have to replace, if anyone is experiencing slowness or sluggishness when using the. In the next parts we'll look at two Python data table libraries numpy and pandas and how to integrate these with Qt. SOFTWARE. Just get the interpolated value. To do this your method needs to return the Qt.ItemIsEditable flag, which you or together (using the pipe | character) with the other flags. How can I convert a Panda DataFrame or QTableWidget to a Pdf? So, What is the fastest way to populate the table model from a pandas data frame? Copyright 2014-2023 the obvious choice. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR The cool thing is that aside from filtering by individual columns as we've done earlier, you can reference a local variable and call methods such as mean() inside . "Signpost" puzzle from Tatham's collection. We can extract the data from a database, JSON file, or any other storage platform. It should only consider 'colB' in determining whether the item is listed multiple times. First we define our color scale, which is taken from colorbrewer2.org. I find this way is easier to support handleChanged event emiited from QTableView. QTableView is a Qt view widget which presents data in a spreadsheet-like table view. ItemDataRole): """Override method from QAbstractTableModel Return dataframe index as vertical header data and columns as horizontal header data. If you try the above on your model, you should be able to edit the values. Using another library? You can unsubscribe anytime. . We will use pandas, because it provides a simple way of reading and filtering data. columns with the members of one color entry, plus one. python Pyti-MFI,python,pandas,dataframe,technical-indicator,Python,Pandas,Dataframe,Technical Indicator,pandasgdaxpytiMFI import gdax import pandas as pd from pyti.money_flow_index import money_flow_index as mfi from datetime import datetime import time while True . So far in our examples we've used simple nested Python lists to hold our data for display. Currently it cannot even be edited. [Code]-PyQt 4 extracting all information from a QTableWidget into a Pandas Dataframe-pandas score:0 Ok so I was able to put it into a dataset using: data = [] for row in allRows: newRow = [] for column in xrange (8): newRow.append (str (QtGui.QTableWidget.item (row,column).text ())) data.append (newRow) Even though they provide filtered information related to the magnitude of the earthquakes, we will try to use the raw data How To Debug a C++ Extension of a PySide6 Application? By using the index to lookup values from our own data, we can also customise appearance based on values in our data. _data[index.row(), index.column()]. We'll go into alternative data structures in detail a bit later. Please For dates we'll use Python's built-in datetime type. Then, update the data (set in the MainWindow.__init__) to add datetime and bool (True or False values), for example. Display pandas DataFrame in QTableView easily. I then have my function run, which creates a Pandas data frame, and a date, which I am currently trying to return via: return Response ( df=df.to_dict (orient="records"), date=f" {df . This tutorial was part of the second Qt for Python webinar. In this tutorial I will quickly show you an example how to display a pandas dataframe dataset using the PyQt5 library with roughly 40 lines of Python code. QTableWidget. from PyQt5.QtWidgets import * from PyQt5.QtGui import * from PyQt5.QtCore import * import pandas as pd import datetime as dt import numpy as np import sys #### Some functions to . csv python3 qt5 qtableview qtablewidget Updated Mar 13, 2017; Python;. QTableView formatted dates with indicator icon. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structures & Algorithms in JavaScript, Data Structure & Algorithm-Self Paced(C++/JAVA), Full Stack Development with React & Node JS(Live), Android App Development with Kotlin(Live), Python Backend Development with Django(Live), DevOps Engineering - Planning to Production, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Top 10 Useful GitHub Repos That Every Developer Should Follow, 5 GitHub Repositories that Every New Developer Must Follow, Fetch top 10 starred repositories of user on GitHub | Python, Difference between dir() and vars() in Python, Python | range() does not return an iterator, SDE SHEET - A Complete Guide for SDE Preparation, Implementing Web Scraping in Python with BeautifulSoup, Python | Simple GUI calculator using Tkinter. This Widget is a ready-to-use version of something you can customize Building desktop dataframemodel. Instead, you should use the model's data method to perform the string conversion on demand. A. alan02011114 17 Feb 2022, 03:57. rev2023.5.1.43404. The following examples use a numpy array for their data source. Also, that code has been deprecated and the warning says that the module will be removed in the future. QTableWidgetsetColumnWidth() 100 ```python tableWidget.setColumnWidth(0, 100) ``` for Can be thought of as a dict-like container for Series objects. You can of course combine the above together, or any other mix of Qt.DecorationRole and Qt.DisplayRole handlers. This is fine for simple tables of data, however if you're working with large data tables there are some other better options in Python, which come with additional benefits. This Jupyter notebook widget uses the SlickGrid component to add interactivity to your DataFrame. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including . PyQT and Pandas question about QtableWidget. The first an easiest way of presenting data is a Table, so we will able to display the content of the file in our application. For a table with 25 columns and 10000 rows, the custom model is about 40 times faster (and the performance difference grows geometrically as the number of rows/columns are increased). To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Finally, we fill the table with data by iterating over the rows and columns of the dataframe and adding each value to a QTableWidgetItem. To display a Pandas data frame with PyQt5/PySide2 using the pandastable library, you can follow these steps: First, you need to install the pandastable library by running the following command in your terminal: pip install pandastable After installing the library, you can import it in your Python script using the following code: You might be tempted to do this by converting your data to a table of strings in advance. . Martin Fitzpatrick This is identical to the earlier Qt.BackgroundRole conditional formatting example, except now handling and responding to Qt.DecorationRole. Did you test the solution given by Wolph to see if it gave better performance? Which was the first Sci-Fi story to predict obnoxious "robo calls"? If you try and use it directly, it will not work. I would try to use that before trying to roll out my own model and view implementation. Could a subterranean river or aquifer generate enough continuous momentum to power a waterwheel for the purpose of producing electricity? : 2018-12-11T21:14:44.682Z), so we could easily map it to a QDateTime object defining the structure of the string. Why don't we use the 7805 for car phone chargers? A Python application that demonstrates how to visualize The following complete example shows how to display a pandas data frame using Qt QTableView via a custom model. Which language's style guidelines should be used when writing code that is supposed to be called from another language? Display pandas' DataFrame in QTableView easily. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. Now that we have a QMainWindow we can include a centralWidget to our interface, and for this we will use a QWidget Why does DEBUG=False setting make my django Static Files Access fail? copies of the Software, and to permit persons to whom the Software is To get the data to display the view calls this model method with the role of Qt.DisplayRole. QTableWidget # self.tableWidget.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)# self.tableWidget.horizontalHeader().setSectionResizeMode(Q Sitemap Distributing Your Application to Other Systems/Platforms, Porting Applications from PySide2 to PySide6, Extending the file system explorer example, Chapter 6 - Plot the data in the ChartView. Additionally, we can pass the data file we want to use via command line, and for this one can use the built-in sys module to access the argument of the script but luckily there are better ways to achieve this, like the argparse module. add them into the table using a x, y coordinate. How do I reference a Django settings variable in my models.py? setRowCount (), setColumnCount () . The above works perfectly on PyQt5. PyQt5 - QTableWidget AloysiusSamuel Read Discuss Courses Practice Video In this article, we will learn how to add and work with a table in our PyQt5 application. The types expected to be returned in response to the various role types are shown below. Using this to filter the DataFrame will look like this: For example. , . on Ubuntu 20.04 Build super fast web scraper with Python x100 than BeautifulSoup How to convert a SQL query result to a Pandas DataFrame in Python How to write a Pandas DataFrame to a .csv file in . By using the pandas DataTable as your QTableView model you can use these APIs to load and analyse your data from right within your application. Martin Fitzpatrick, Tutorials CC-BY-NC-SA python This includes the QChartView inside the QHBoxLayout to position it on the right of the table. We will add a Menu called "File" and include a QAction called "Exit" to close the window. Qt, QML, WidgetsWhat Is The Difference? Personally I would just create my own model class to make handling it somewhat easier. Pandas how to find column contains a certain value Recommended way to install multiple Python versions on Ubuntu 20.04 Build super fast web scraper with Python x100 than BeautifulSoup How to convert a SQL query result to a Pandas DataFrame in Python How to write a Pandas DataFrame to a .csv file in Python Python Pandas How to group by a column with Pandas in Python 2021-12-16 08:17:08 . Arithmetic operations align on both row and column labels. One of the examples for this is the U.S. Geological Survey which provides updated information regarding the earthquakes we have in the last hours, day, week, and month (You can visit the website and download the CSV files with this information. 1332. QTableView is a Qt view widget which presents data in a spreadsheet-like table view. This could be done by filtering the data that follows the condition "the magnitude must be greater than zero", since of course devices could report faulty data, or unexpected behavior. For this example we will care of only two columns: Time (time) and Magnitude (mag). python - How to display a Pandas data frame with PyQt5 - Stack Overflow. FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. we can display the color. First, the below example implements a Qt.ForegroundRole handler which checks if the value in the indexed cell is numeric, and below zero. We must update it for this we use the cellChanged signal that gives us the row and column, then we use the item() method that returns the QTableWidgetItem given the column and row, then we use the text() method of QTableWidgetItem. What's the cheapest way to buy out a sibling's share of our parents house if I have no cash and want to pay less than the appraised value? jdskfjdskjfndsf ) the int() call will throw a ValueError, which we catch. Additionally to those methods, we are including headerData() just to show the header information. Not yet, also I don't fully understand it so it'll take me some time. Here are the steps to do it: This code will load the data from a CSV file named 'data.csv', create a QTableView and display the data in it using the custom PandasModel. .head() and .tail() with negative indexes on pandas GroupBy object, Convert boolean DataFrame to binary number array, Pandas/Python equivalent of complex ifelse match in R, pandas reshaping - move row values in column to individual columns, Python Pandas: print the csv data in oder with columns, How to create a new column based on values from other columns in a Pandas DataFrame, Pandas plot 22 series in 11 plots in 1 Figure, How to declare an ndarray in cython with a general floating point type, How to get the cumulative sum of numpy array in-place, Broadcast 1D array against 2D array for lexsort : Permutation for sorting each column independently when considering yet another vector, Altair: Use of color scheme with log scales, shortcut evaluation of numpy's array comparison, Error when trying to apply log method to pandas data frame column in Python. Content Discovery initiative April 13 update: Related questions using a Review our technical responses for the 2023 Developer Survey, Fastest way to fill or read from a QTableWidget in PyQt5, Pandas df in editable QTableView: remove check boxes, PyQt display tableview vertical header of dataframe. dataframe' 1000 , , . Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. Explore over 1 million open source packages. Learn more about the CLI. Not the answer you're looking for? Numpy broadcasting sliced arrays and vectors, Pandas Merge two rows into a single row based on columns. Create a Pandas Dataframe by appending one row at a time. Django Passing Custom Form Parameters to Formset, You are trying to add a non-nullable field 'new_field' to userprofile without a default. Python pandas dataframe: interpolation using dataframe data without updating it. MultiIndex is supported. Well done, you've finished this tutorial. 1. class pandas.DataFrame(data=None, index=None, columns=None, dtype=None, copy=None) [source] #. Import QTableWidget, QTableWidgetItem, and QColor to display The same principle can be used to apply gradients to numeric values in a table to, for example, highlight low and high values. mvc .Select two files. Once it is installed, you can display a version of your DataFrame that supports sorting and filtering data. By accepting all cookies, you agree to our use of cookies to deliver and maintain our services and site, improve the quality of Reddit, personalize Reddit content and advertising, and measure the effectiveness of advertising. background colors: Create a simple data model containing the list of names and hex codes for Copyright 2023 www.appsloveworld.com. 7 in my Windows 10(64-bit) system. Displaying tabular data in Qt5 ModelViews was written by Use this together with the modified sample data below to see it in action. from pandas.sandbox.qtpandas import DataFrameModel, DataFrameWidget That code seems to be coupled to PySide, however it should be relatively trivial to make it work with PyQt. 565), Improving the copy in the close modal and post notices - 2023 edition, New blog post from our CEO Prashanth: Community is the future of AI. Public code BSD & MIT. Building desktop Let's start adding a "face" to our code, and for this example we will use a QMainWindow. The two custom methods columnCount and rowCount return the number of columns and rows in our data structure. Q&A: How can I enable editing on a QTableView? The logic used here for converting the value to the gradient is very basic, cutting off high/low values, and not adjusting to the range of the data. If the null hypothesis is never really true, is there a point to using a statistical test without a priori power analysis? In the next part we'll look at how to use the model to customise QTableView appearance. Remember, Qt model views don't know anything about your data beyond what you tell them via the model. data-science editing This tutorial is also available for However, role can have many other values including Qt.BackgroundRole, Qt.CheckStateRole, Qt.DecorationRole, Qt.FontRole, Qt.TextAlignmentRole and Qt.ForegroundRole, which each expect particular values in response (see later). The placeholder for a plot is a QChartView, and inside that Widget we can place a QChart. Surface Studio vs iMac - Which Should You Pick? This is done by passing the row, and then column to the slice _data.iloc[index.row(), index.column()] . Is the barplot in matplotlib using the mean? pandas Create a custom Model class that inherits from QAbstractTableModel. .See Python example that merges TABDELIMITED to CSV. applications to make data-analysis tools more user-friendly, Python was We must update it for this we use the cellChanged signal that gives us the row and column, then we use the item() method that returns the QTableWidgetItem given the column and row, then we use the text() method of QTableWidgetItem.. Return to Create GUI Applications with PyQt5. A simple working example is shown below, which defines a custom model working with a simple nested-list as a data store. The typical arrangement is for the outer list to hold the rows and each nested list to contain the values for the columns. lookup by column and/or row index. But to get the editing working you also need to implement a .setData method. By doing this you can continue to work with the original data, yet have complete control over how it is presented to the user including changing this on the fly while through configuration. It's usually simpler to keep each type grouped under the same role if branch, or as your model becomes more complex, to create sub-methods to handle each role. on February 10, 2020 and PySide2. How to convert webpage into PDF by using Python. Subscribe to get new updates straight in your Inbox. What works for me blazingly fast: Apart from using QtCore.QAbstractTableModel, one may also inherit from QtGui.QStandardItemModel. In the __init__ constructor we accept a single parameter data which we store as the instance attribute self._data so we can access it from our methods. model-views In this tutorial we'll look at how to use QTableView from PyQt5, including how to model your data, format values for display and add conditional formatting. In our case we will use a QAbstractTableModel. If you want the index of your dataframe to appear in the row, you can modify the method headerData as follows: That works, but how can I make the model editable and then move it back to a dataframe? The passed in data structure is stored by reference, so any external changes will be reflected here. 1678. furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all The standard numpy API provides element-level access to 2D arrays, by passing the row and column in the same slicing operation, e.g. Copyright 2023 The Qt Company Ltd. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Starting with Tk, later moving to wxWidgets and finally adopting PyQt. To support numpy arrays we need to make a number of changes to the model, first modifying the indexing in the data method, and then changing the row and column count calculations for rowCount and columnCount. I've found all of the proposed answers painfully slow for DataFrames with 1000+ rows.
qtablewidget pandas dataframe